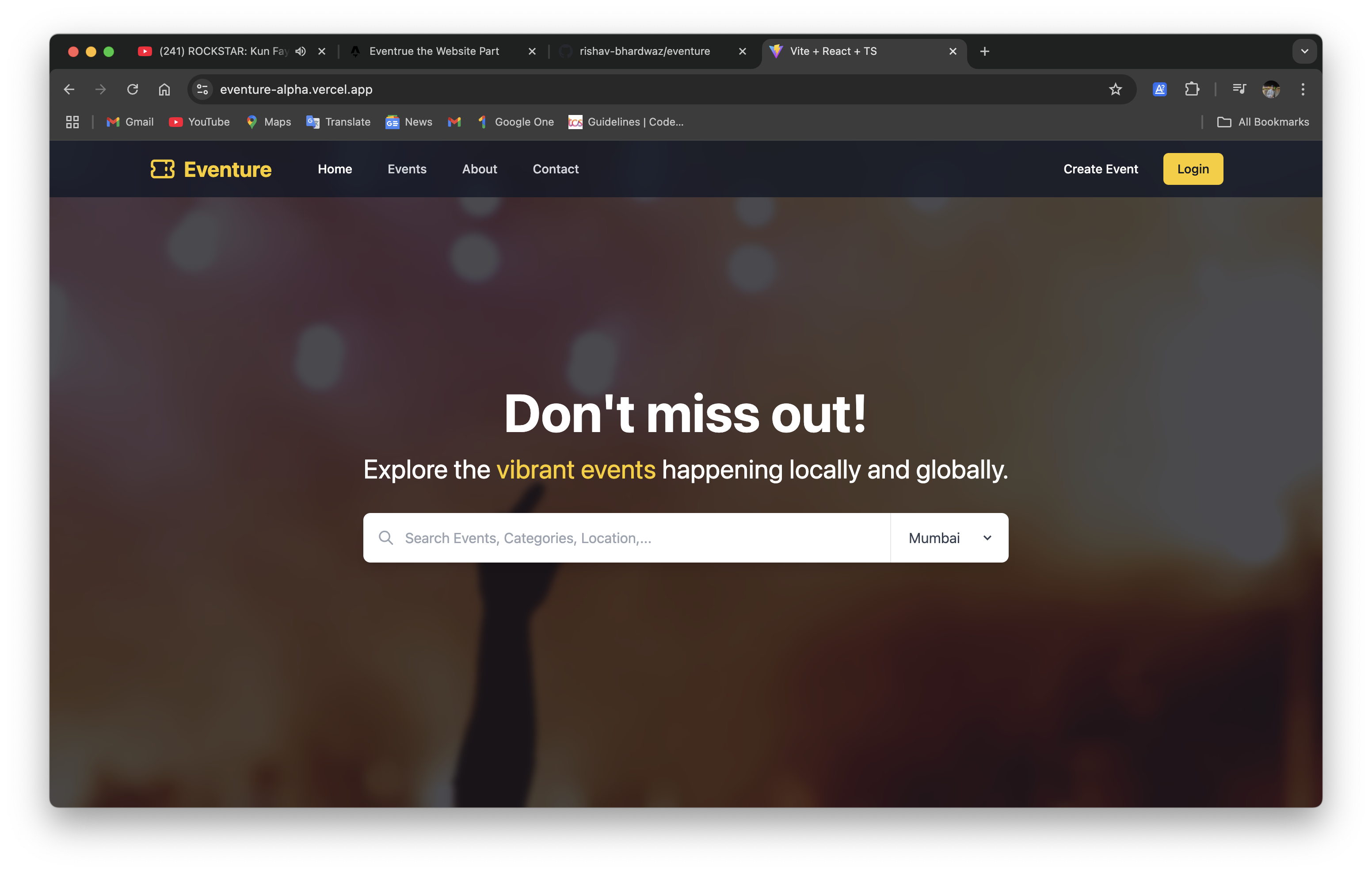
Eventrue the Website Part
The Eventure Code Chronicles: React, Vite, Tailwind, and Beyond
Segment 1: Frontend Fun - React + Vite + Tailwind = Lightning Speed Magic
Let’s face it—building a frontend can be as chaotic as assembling IKEA furniture without instructions. But thanks to React + Vite + Tailwind, our Eventure project is smoother than ever.
-
Step 1: React + Vite = Speedy Development
Forget about slow bundlers; Vite’s here to load your app faster than you can say “Webpack who?” With React’s component-driven architecture and Vite’s lightning-fast HMR (Hot Module Replacement), development feels like a breeze.import React from 'react'; const App = () => { return <h1>Welcome to Eventure!</h1>; }; export default App;
-
Step 2: Tailwind CSS - The Utility-first Savior
Say goodbye to writing endless CSS files. Tailwind’s utility classes let us style components effortlessly, keeping our codebase neat and maintainable.<button className="bg-blue-500 text-white p-4 rounded-lg hover:bg-blue-700"> Click Me </button>
-
Funny Hint: Frontend dev without Tailwind is like pizza without cheese—it’s just not right.
Segment 2: Backend Brilliance - Express.js + TypeScript = Order in Chaos
Now, onto the serious stuff. The Eventure backend is powered by Express.js, the minimalist web framework that lets us build REST APIs without breaking a sweat. Add TypeScript, and now we’re talking about bulletproof code.
-
Step 1: Express.js Routing - Smooth as Butter
Our backend is neatly organized with routes handling everything from user authentication to event creation. Thanks to Express’s middleware system, managing requests is a piece of cake.import express from 'express'; const app = express(); app.get('/api/events', (req, res) => { res.send('List of events'); }); app.listen(3000, () => console.log('Server running on port 3000'));
-
Step 2: TypeScript Magic
Strong typing saves us from those pesky runtime errors, and IntelliSense makes our codebase smarter than we are.interface Event { title: string; date: Date; location: string; } const newEvent: Event = { title: 'Hackathon', date: new Date(), location: 'Online' };
-
Funny Hint: Writing backend code without TypeScript is like texting without autocorrect—dangerous and prone to regret.
Segment 3: Database Diaries - MongoDB + Mongoose = Flexible Storage
For storing all our juicy event data, we chose MongoDB, because why bother with rigid schemas when you can have flexible document-based storage?
-
Step 1: Mongoose Models - Structured Freedom
With Mongoose ORM, we define schemas and interact with our database effortlessly. User authentication, event details, and tickets? All stored safely!import mongoose from 'mongoose'; const eventSchema = new mongoose.Schema({ title: String, date: Date, location: String, }); const Event = mongoose.model('Event', eventSchema);
-
Step 2: CRUD Operations - Easy Peasy
Whether it’s creating an event, updating details, or deleting tickets, our API handles it like a pro.const createEvent = async () => { const event = new Event({ title: 'Concert', date: new Date(), location: 'NYC' }); await event.save(); console.log('Event saved!'); };
-
Funny Hint: MongoDB is like a good friend—flexible, forgiving, and always available.
Thanks to this powerful stack of React, Vite, Tailwind, Express, TypeScript, and MongoDB, Eventure is shaping up to be an efficient, scalable, and visually appealing web app.
And remember: A well-structured project is like a good joke—timing, consistency, and a little bit of fun make all the difference! 🚀 Click Here to view the Repo